Swagger는 개발한 Rest API를 편리하게 문서화해주고, 이를 통해서 관리 및 제3의 사용자가 편리하게 API를 호출해 보고 테스트할 수 있는 OpenApi에서 만든 양식이다.
OpenAPI Specification - Version 3.1.0 | Swagger
OpenAPI Specification - Version 3.1.0 | Swagger
swagger.io
일반적으로 굉장히 많이 쓰이고 이를 통해 클라이언트, 모바일 개발자 분들과 소통하며 개발을 이어나가곤 한다. 그래서 Swagger는 서버 개발자의 의도를 명확하게 나타내야 한다.
이에 따라 각각 서버에서 사용하는 양식들은 굉장히 많다. 대표적으로 REST Docs와 Swagger annotation으로 나타내는 경우가 많다.
문제
그 중 살펴볼 건은 지금 현재 사용 중인 Swagger 어노테이션을 통해 컨트롤러 API에 이를 나타낸 것이다. 아래를 먼저 보자.
@ApiResponses(
value = {
@ApiResponse(
responseCode = "201",
description = "이전까지 회원가입을 하지 않았던 경우",
content = @Content(
schema = @Schema(implementation = ApiSuccessResponseDto.class)))
@ApiResponse(
responseCode = "200",
description = "이미 회원가입을 했던 유저인 경우",
content = @Content(
schema = @Schema(implementation = ApiSuccessResponseDto.class)))
})
만약 여기서 여러가지 Validation이 필요한 경우 적절한 Status Code와 메시지를 통해 이를 노출시켜줘야 한다. 하지만 조금만 상상해 보자. 400번대가 만약 5개 이상이 넘어가는 경우..? 이는 곧 엄청난 코드량을 포함하고 있을 것이다. (실제로 그렇다)
그렇다면 어떻게 하면 이를 구분하고 편하게 스웨거에도 명시적으로 표현해줄 수 있을까? 거기에 더해 시큐리티의 인증 여부도 같이 넣어줄 수 있을까? 그리고 같은 Status Code일 때도 명시적으로 나타내줄 수 없을까?
⇒ 이에 대한 해답으로 나는 Security Config와 Swagger Config를 통해 애플리케이션이 로딩될 때를 기점으로 해결했다.
또한, Swagger에 변동사항이 생기면 ci단에서 자동으로 이를 인식하여 (왜냐하면 이것은 곧 json Schema 이기 때문) 해결하였다.
⇒ 더하여, Custom Annotation을 만들어서 우리가 정의한 ErrorTitle을 조금 더 쉽게 기술할 수 있도록 만들었다.
최종 결과를 먼저 확인 해보자.
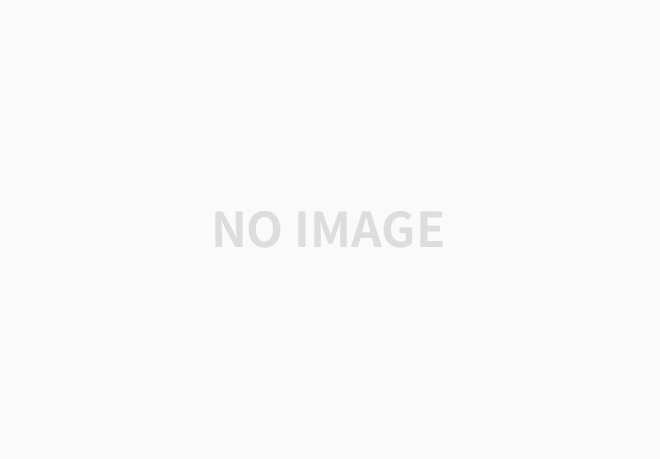
@XXPostMapping("[EXAMPLEURL]", authenticated = true or false, role = [ROLE_NAME])
@SecurityRequirement(name = "Bearer Authentication")
@ApiResponse(responseCode = "200", description = "Message")
@CustomFailResponseAnnotation(exception = ErrorTitle.NotFoundUserReward)
@CustomFailResponseAnnotation(exception = ErrorTitle.AlreadyReceivedReward)
....
fun ...method()
여기서 나타내는 XXPostMapping은 해당 Custom Annotation으로 인증 여부, 권한 여부 등을 핸들링할 수 있는 부분이다.
또한 CustomFailResponseAnnotation은 스웨거에서 표현해 주는 여러 가지 에러들을 손쉽게 정의한 ErrorTitle에 맞춰 넣어주기만 한다면 스웨거에서 이를 자동으로 표현하게 해 준다.
맨 처음 보았던 것과 코드 양에서 큰 차이가 난다.
해결 방법
먼저 커스텀하게 예외를 보여주는 부분은 아래 OpenApi 응답을 커스텀하게 변경해야 한다.
OpenAPI-Specification/versions/3.1.0.md at 3.1.0 · OAI/OpenAPI-Specification
OpenAPI-Specification/versions/3.1.0.md at 3.1.0 · OAI/OpenAPI-Specification
The OpenAPI Specification Repository. Contribute to OAI/OpenAPI-Specification development by creating an account on GitHub.
github.com
// Operation 중 responses 필드
"responses": {
"200": { // Response
"description": "Pet updated.",
"content": {
"application/json": {},
"application/xml": {}
}
},
"405": { // Response
"description": "Method Not Allowed",
"content": {
"application/json": {},
"application/xml": {}
}
}
},
즉, 공식문서에서 설명하고 있는 responses안에 있는 Response 객체들을 커스텀하게 우리 형식에 맞게 바꿔 쳐 줘야 한다.
⇒ 결론적으로 내가 해결한 방법은 Custom Annotation을 활용해 이를 인식하도록 하여 Operation의 response 객체를 바꿔주는 형식이다. Swagger springdoc에서 제공하는 OperationCustomizer
를 커스텀하게 받아서 코드를 구성한다면 간편하게 해결할 수 있다.
⇒ 추가적으로 보아야 할 것은 content 안에 application/json 형식 안에 있는 객체는 Media Type Object이다. 아래 공식문서에 확인 가능하며 더 살펴보자면 examplse 안에는 Example Object가 올 수 있는데 우리가 정의한 값과 에러 code를 품고 있는 에러 타이틀로 이를 가져와서 적어주는 형식이다.
OpenAPI-Specification/versions/3.1.0.md at 3.1.0 · OAI/OpenAPI-Specification
OpenAPI-Specification/versions/3.1.0.md at 3.1.0 · OAI/OpenAPI-Specification
The OpenAPI Specification Repository. Contribute to OAI/OpenAPI-Specification development by creating an account on GitHub.
github.com
// in CustomOperationCustomizer : OperationCustomizer
override fun customize(operation: Operation, handlerMethod: HandlerMethod): Operation {
val methodAnnotations = handlerMethod.method.declaredAnnotations
val responses = operation.responses
if(methodAnnotations.find { it is Hidden } != null){
return operation
}
for (annotation in methodAnnotations) {
when (annotation) {
is CustomFailResponseAnnotations -> {
for (j in annotation.value) {
val message = if (j.message == "") j.exception.message else j.message
handleCustomFailResponse(j.exception, message, responses)
}
}
is CustomFailResponseAnnotation -> {
val message = if (annotation.message == "") annotation.exception.message else annotation.message
handleCustomFailResponse(annotation.exception, message, responses)
}
}
....
}
}
...
fun handleCustomFailResponse(
exception: ErrorTitle, // 필자가 정의한 공용 에러 타이틀
message: String?,
responses: ApiResponses
) {
val statusCode = exception.status.value().toString()
val response = responses.computeIfAbsent(statusCode) { ApiResponse() }
val content = response.content ?: Content()
val schema = Schema<Any>().`$ref`("#/components/schemas/필자가 사용한 공용 실패 응답 모델")
val errorResponse = 필자가 사용한 공용 실패 응답 모델
val mediaType = content.getOrPut("application/json") { MediaType().schema(schema) }
val example = Example().value(errorResponse)
mediaType.addExamples(errorResponse.message, example)
content["application/json"] = mediaType
response.content(content)
responses.addApiResponse(statusCode, response)
}
// in Annotation
@Target(AnnotationTarget.FUNCTION, AnnotationTarget.TYPE, AnnotationTarget.ANNOTATION_CLASS)
@Retention(AnnotationRetention.RUNTIME)
@Inherited
annotation class CustomFailResponseAnnotations(vararg val value: CustomFailResponseAnnotation = []
)
@Target(AnnotationTarget.FUNCTION, AnnotationTarget.TYPE, AnnotationTarget.ANNOTATION_CLASS)
@Retention(AnnotationRetention.RUNTIME)
@JvmRepeatable(CustomFailResponseAnnotations::class)
annotation class CustomFailResponseAnnotation(val exception: ErrorTitle, val message: String = "")
코드를 살펴보자.
val statusCode = exception.status.value().toString()
- 예외의 HTTP 상태 코드를 문자열로 가져옵니다
val response = responses.computeIfAbsent(statusCode) { ApiResponse() }
- 해당 상태 코드에 대한 ApiResponse 객체를 가져오거나, 없으면 새로 생성합니다
val content = response.content ?: Content()
- response의 content를 가져오거나, null이면 새로운 Content 객체를 생성합니다
val schema = Schema<Any>().
$ref("#/components/schemas/필자가 사용한 공용 실패 응답 모델")
- 필자가 사용한 공용 실패 응답 모델을 참조하는 스키마를 정의합니다
val errorResponse =
필자가 사용한 공용 실패 응답 모델- 실제 에러 응답 객체를 생성합니다
val mediaType = content.getOrPut("application/json") { MediaType().schema(schema) }
- application/json MediaType 객체를 가져오거나 새로 생성하고 스키마를 설정합니다
val example = Example().value(errorResponse)
- errorResponse를 포함하는 새로운 Example 객체를 생성합니다
mediaType.addExamples(errorResponse.message, example)
- MediaType에 예시를 추가합니다. 키는 에러 메시지를 사용합니다
content["application/json"] = mediaType
- 변경된 MediaType을 content에 다시 설정합니다
response.content(content)
- 응답에 변경된 content를 설정합니다
responses.addApiResponse(statusCode, response)
- 최종적으로 상태 코드에 대한 응답을 Swagger responses에 추가합니다
위와 같이 설정하면 우리가 정의한 CustomFailResponseAnnotations
혹은CustomFailResponseAnnotation
을 활용하여 이를 핸들링 하여 OpenAPI에서 제공하는 객체들을 조작하여 나타낼 수 있는 것이다.
이렇게 CustomOperationCustomizer를 만들고 아래처럼 스웨거를 빌드할 때 등록만 해준다면 우리가 원하는 결과를 볼 수 있다!
// in Swagger Build
return GroupedOpenApi.builder()
.group("API")
.pathsToMatch("/**")
.addOpenApiCustomizer(customOpenApiCustomizer)
.addOperationCustomizer(customOperationCustomizer)
.build()
또 다른 문제인 인증, 권한
필자는 Spring Security를 통해 applicationContext를 기반으로 Spring Security의 설정을 동적으로 구성하여 컨트롤러들을 스캔하여 특정 어노테이션이 붙은 엔드포인트에 대한 권한 설정을 자동화하는 방향을 구성했다.
⇒ 이는 애플리케이션의 실행시점에 모든 것을 알 수 있기 때문이다.
// 시큐리티 설정
fun filterChain(http: HttpSecurity) = http
...
.applyDynamicUrlSecurity(applicationContext)
...
// 확장함수
fun HttpSecurity.applyDynamicUrlSecurity(applicationContext: ApplicationContext): HttpSecurity {
...
}
위를 통해 동적으로 특정 Api Url에 응답을 설정할 수 있는 것이다. 조금 더 자세히 살펴보자!
val controllers: Map<String, Any> = applicationContext.getBeansWithAnnotation(
Controller::class.java
)
for (controller in controllers.values) {
var parentPath: String? = null
val methods = controller.javaClass.declaredMethods
val requestMapping = controller.javaClass.getAnnotation(RequestMapping::class.java)
if (requestMapping != null && requestMapping.value.isNotEmpty()) {
parentPath = requestMapping.value[0]
}
for (method in methods) {
if (method.isAnnotationPresent(XXGetMapping::class.java)) {
val XXGetMapping = method.getAnnotation(XXGetMapping::class.java)
val paths = XXGetMapping.value
if (XXGetMapping.hasRole.isNotEmpty()) {
this.authorizeHttpRequests {
if (paths.isEmpty()) {
it.requestMatchers(HttpMethod.GET, parentPath).hasAnyRole(*XXGetMapping.hasRole)
}
paths.forEach { p ->
var path = if (!p.startsWith("/")) "/$p" else p
path = if (parentPath == null || parentPath == "null") p else parentPath + path
it.requestMatchers(HttpMethod.GET, path).hasAnyRole(*XXGetMapping.hasRole)
}
}
} else if (XXGetMapping.authenticated) {
this.authorizeHttpRequests {
if (paths.isEmpty()) {
it.requestMatchers(HttpMethod.GET, parentPath).authenticated()
}
paths.forEach { p ->
var path = if (!p.startsWith("/")) "/$p" else p
path = if (parentPath == null || parentPath == "null") p else parentPath + path
it.requestMatchers(HttpMethod.GET, path).authenticated()
}
}
}
}
}
}
위 코드 구성을 살펴보면 대략 흐름은 아래와 같다.
- 리플렉션을 사용하여
@Controller
어노테이션이 붙은 모든 빈들을 찾는다.- Spring의 ApplicationContext를 통해 수행된다.
- 컨트롤러 클래스에서
@RequestMapping
annotation을 찾는다. javaClass
를 통해 클래스의 메타데이터에 접근한다.- 리플렉션을 사용하여 컨트롤러 클래스의 모든 선언된 메서드를 가져온다.
- 리플렉션을 사용하여 메서드에
@XXGetMapping
어노테이션이 있는지 확인한다. - 이후 authenticated 여부를 통해동적으로 이에 대한 인증 여부 및 권한 여부를 넣어준다.
위 같이 구성하면 동적으로 annotation을 통해 우리가 원하는 값들을 넣어줄 수 있다.
여기서는 XXGetMapping
만 다루었지만 다른 매핑들도 비슷한 형태로 구현할 수 있다. 그리고 이를 Swagger에도 녹여줄 것이다.
XXGetMapping -> {
if(annotation.authenticated || annotation.hasRole.isNotEmpty()){
operation.addSecurityItem(SecurityRequirement().addList("Bearer Authentication"))
}
}
위 같이 추가해 준다면 문제없이 스웨거에서도 토큰을 통해 인증을 테스트할 수 있도록 만들 수 있다!!
결론
오늘 기술한 내용은 협업에 있어서 중요한 부분이라고 생각한다. 클라이언트나 모바일 개발자들은 모두 구두를 통한 소통 혹은 스웨거를 통한 소통을 통해 작업이 이루어진다. 그렇기 때문에 정확한 스웨거를 제공하는 것도 백엔드 개발자로서의 중요한 소통 방식이기 때문에 무시할 수 없다.
물론 필자가 구현한 방법이 명확한 해답이 아닐 수 있다. 이는 Application이 가진 컨트롤러 annotation이 많을수록 애플리케이션 구동이 오래 걸릴 수 있다. 하지만 필자는 이를 테스트해본 결과 크리티컬하게 느껴지지 않았다. 이에 대한 느낌보다는 양질의 스웨거와 코드를 작성할 수 있다는 장점이 더 큰 것 같다.
그리고 이게 매우 느리다면 캐싱하는 방식도 생각해 볼 수 있다. 따라서 방법은 많다는 것이다.
'서버 개발(생각과 구현)' 카테고리의 다른 글
보여지는 시간대(타임존)은 누구의 책임인가 (0) | 2025.01.03 |
---|---|
단일 프로젝트 구조와 멀티 모듈 구조 (0) | 2025.01.01 |
JPA N+1의 의도와 문제 해결 (0) | 2024.12.26 |
외부 API와 트랜잭션의 관계를 조심하자 (0) | 2024.12.23 |
락의 필요성과 실제 사용을 위한 분산 락, 낙관적 락, 비관적 락 탐구 (0) | 2024.12.22 |